Turing Machine
The purpose of the theory of Turing machines is to prove that whether a certain specific language have algorithmic solution or not. We will introduce the concept of Turing machines and their properties.
Definition of Turing Machine
We first have a look at the picture of a Turing machine.
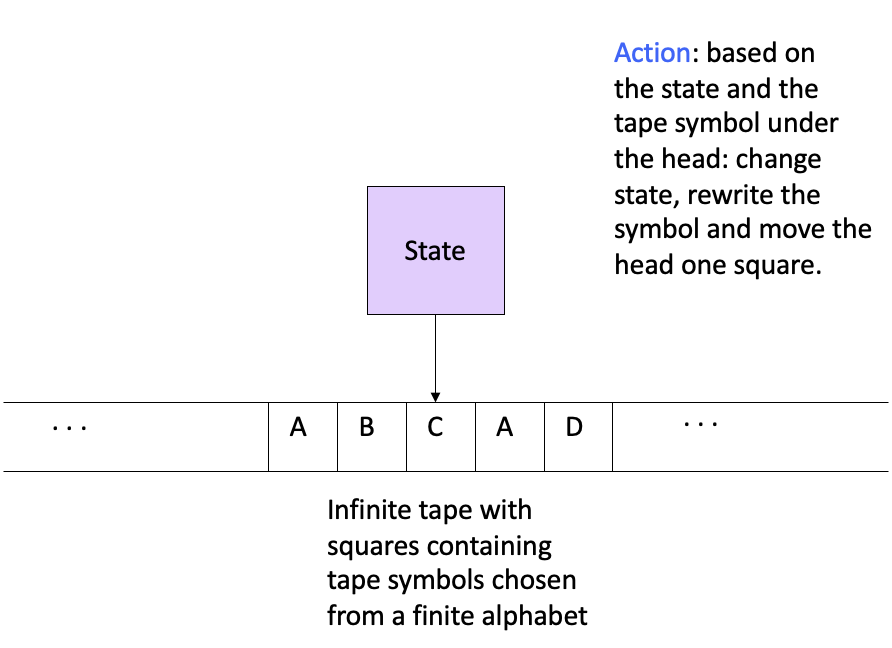
A Turing Machine
We have a tape and a head that can move left or right on the tape. The tape, which is divided into cells, is infinite in both directions. The head itself has a finite number of states, which can change based on the current state and the symbol it reads on the tape. The head can also write symbols on the tape based on its state.
A Turing machine can be formally defined as a 7-tuple
is a finite set of states. is the input alphabet, which does not contain the blank symbol . is the tape alphabet, which contains and the blank symbol . is the transition function. is the start state. is the accept state. is the reject state.
Why Turing Machine?
Why not we just use C program or Python program? The answer is that you can, but it is easier to prove things with Turing machines. The Turing machine is a theoretical model of computation, which is simple and powerful. What's more, it is equivalent to any other model of computation and its memory is free of charge!
Transition Function
The transition function
For example, if the Turing machine is in state
Instantaneous Description
We can also take a photo of the Turing machine at a certain moment, which is called the instantaneous description. It is denoted as
Then we can have the following transition:
- If
, then . - If
, then . (Of course, )
We can also define
Language of a Turing Machine
A Turing machine
Do we have to eat the whole string?
We don't need to consume the whole input string in a Turing machine to accept it. The Turing machine can accept the string by halting or reaching final state at any moment. This is different from the PDA, which must consume the whole string to accept it.
Equivalence of Acceptance
We can prove that each
Proof
( From
( From
Recursive and Recursively Enumerable Languages
The Turing machine can accept languages in two ways: by final state or by halting. And we now know the two language are equivalent. Thus, we define this set of languages as recursively enumerable languages. This term has a long history and don't be confused by the name. Almost all languages we can think of are recursively enumerable.
An algorithm
If
We can conclude that:
- Recursive: Accept (string in the language) or Reject (string not in the language). (Or, Halt or Loop)
- Recursively Enumerable: Accept (string in the language), Reject or Loop (string not in the language).
Closure Properties
Both recursive and recursively enumerable languages have the following closure properties:
- Union (Easy to show): If
and are recursive (or recursively enumerable), then is recursive (or recursively enumerable). - Intersection (Easy to show): If
and are recursive (or recursively enumerable), then is recursive (or recursively enumerable). - Concatenation: If
and are recursive (or recursively enumerable), then is recursive (or recursively enumerable). - Kleene Star: If
is recursive (or recursively enumerable), then is recursive (or recursively enumerable). - Reverse: If
is recursive (or recursively enumerable), then is recursive (or recursively enumerable). - Inverse Homomorphism: If
is recursive (or recursively enumerable), then is recursive (or recursively enumerable), where is a homomorphism.
Proof of Closure Properties
Concatenation: For recursive enumerable language
Reverse: We can first reverse the input string and run the Turing machine on it.
Only recursive languages have the following closure properties:
- Difference: If
and are recursive, then is recursive. - Complement: If
is recursive, then is recursive.
No complement in RE
If
However, the complement of RE is called
But RE is under the closure of homomorphism.
No homomorphism in recursive languages
Consider a Turing machine whose language
Read more here.
Turing Machine Programming
We know that recursively enumerable languages are powerful, so is the Turing machine. Generally, unlike former DFA or PDA, Turing machine is not used to recognize languages, but to compute functions, which is related to programming.
Let's have a look at the following example:
Example 1 Construct a Turing Machine to recognize the language
Solution 1 We can construct a Turing machine as follows:
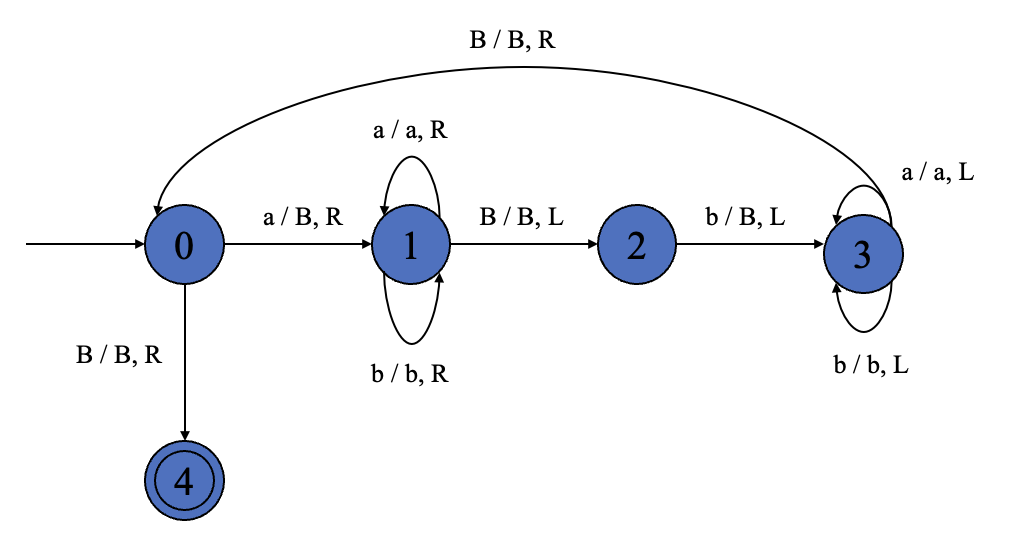
The graph of solution 1
The transitions are shown on the graph above. Note that the sign
Suppose the input string is
while( S != "" ) {
if(S[0] == 'a') {
S.erase(0, 1);
}else{
reject;
}
if(S[S.size()-1] == 'b') {
S.erase(S.size()-1, 1);
}else{
reject;
}
}
accept;
2
3
4
5
6
7
8
9
10
11
12
13
Example 2 Construct a Turing Machine to shift each input symbol one cell to the right, while the leftmost symbol is replaced by
Solution 2 We can construct a Turing machine as follows:
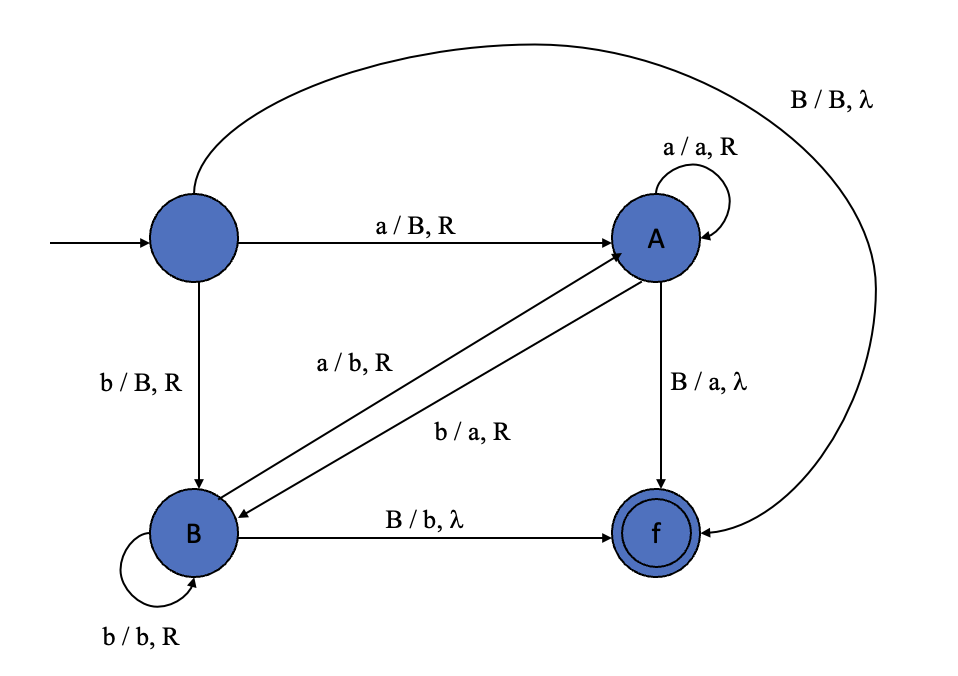
The graph of solution 2
Example 3 Construct a Turing Machine to shift its words cyclically to the right.
Solution 3 We can construct a Turing machine as follows:
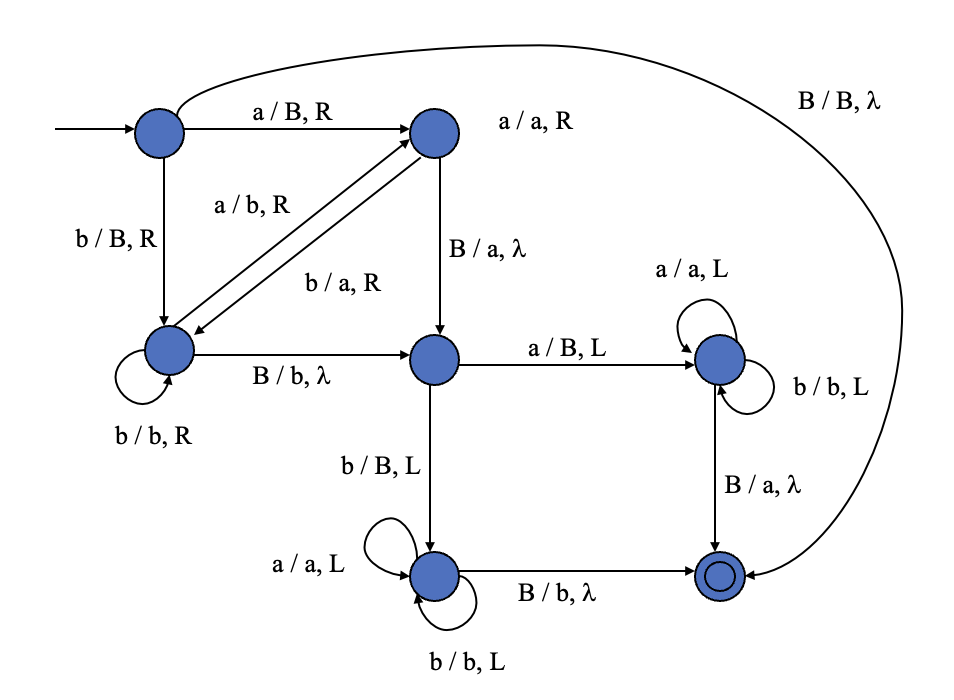
The graph of solution 2
Note that the upper-left corner of the graph is the same as the graph in solution 2, and the lower-right corner is to write the last symbol to the first cell. We can perceive this idea of constructing turing machine as structured programming.
Example 4 (Decider) Let
Solution 4 Trivially, we can construct a Turing machine without decider as follows:
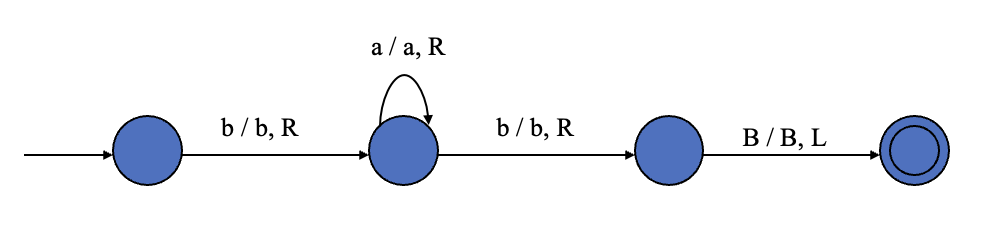
A prototype of solution 4
Then, we can add a decider to the Turing machine as shown below:
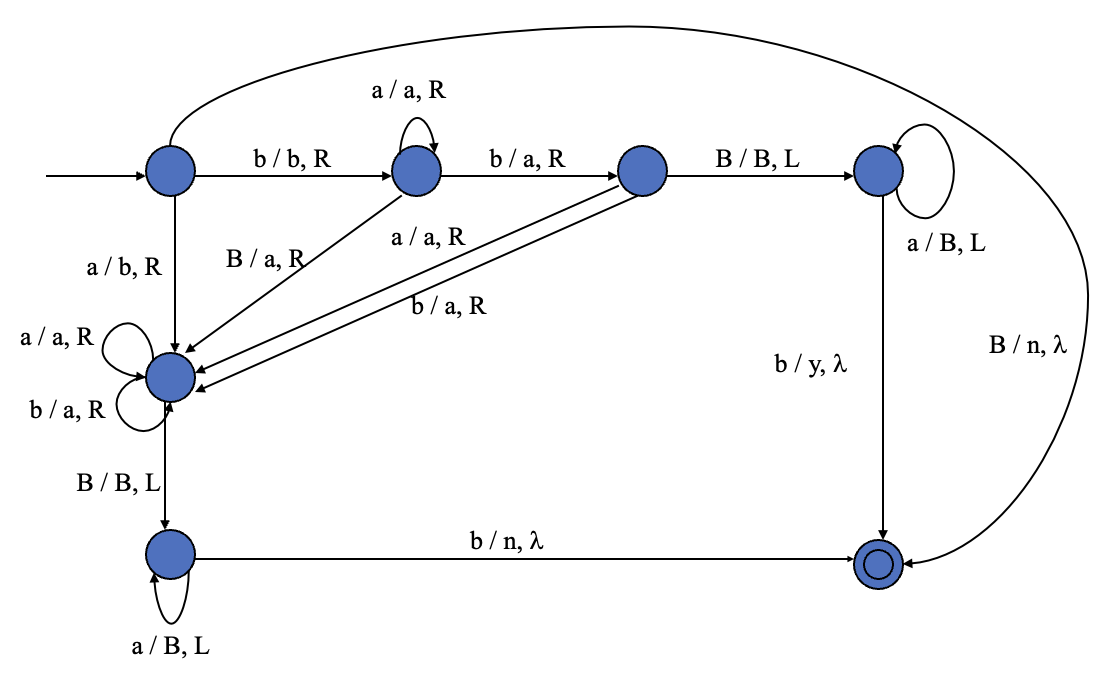
The graph of solution 4
The decider makes its way to the leftmost cell, modifying all the cells to blank symbols along the way, then subsequently writes
Programming Tricks
Multiple Tracks
We can use the standard Turing machine to simulate a multi-track Turing machine. The machine still possesses only one tape, but each cell can store multiple symbols, as vector (or tuple, whatever you like). The head can read and write multiple symbols at once.
We can use the symbol
Marker
Using the trick of multiple tracks, we can make one extra track the marker track. Almost all the cells on the marker track are blank symbols, but few are marked with a special symbol (Marker) to indicate some information.
Extensions
There are many extensions of Turing machines, which are more general than the standard Turing machine. However, they are all equivalent to the standard Turing machine, but more intuitive and easier to use. Here are some of them:
- Semi-infinite Turing machine
- Multi-tape Turing machine
- Non-deterministic Turing machine
Semi-infinite Turing Machine
A semi-infinite Turing machine has a semi-infinite tape, which is infinite in one direction (usually right) and finite in the other.
We can use multi-track Turing Machine to simulate it. Just imagine the infinite track being folded into a semi-infinite tape, denoting by one upper track and lower track. The machine can remember whether simulating the upper track or the lower track by the state. If simulating the lower track, the head moves the opposite direction to that in the previous infinite tape. If the head reaches the leftmost cell, it shifts its state to simulate the other track.
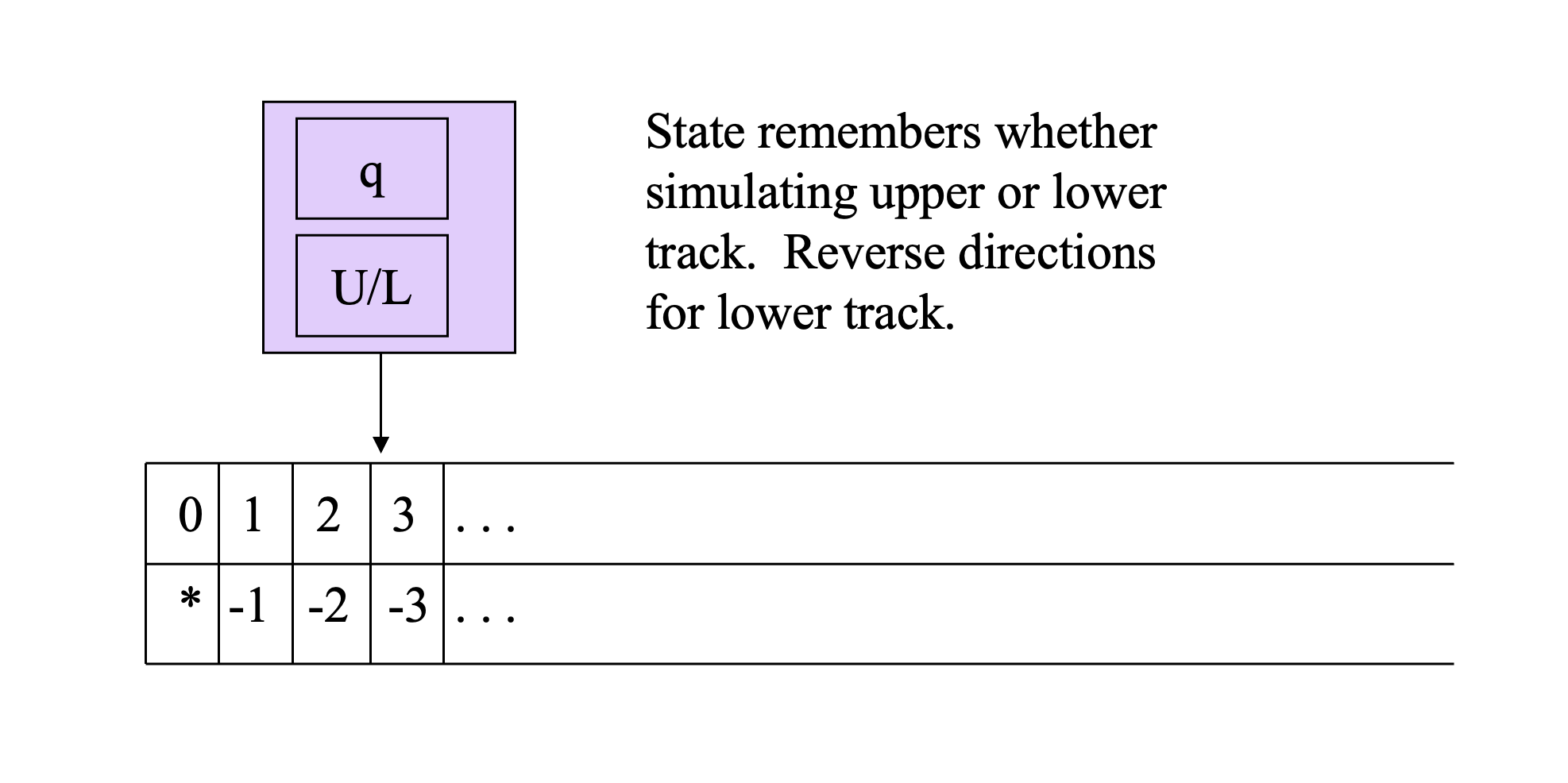
Simulating Semi-infinite TM
What about two stacks?
The semi-infinite tape is just like two stacks. Actually, we can even just use two stacks to simulate Turing machines.
We know, the ID of a Turing machine is
Multi-tape Turing Machine
Difference between Multi-tape and Multi-track
The multi-tape Turing machine has multiple tapes, and the heads can move independently. The multi-track Turing machine has only one tape, but each cell can store multiple symbols.
We can show that all multi-tape Turing machines can be simulated by a single-tape & multi-track Turing machine. A simple idea is that: for a
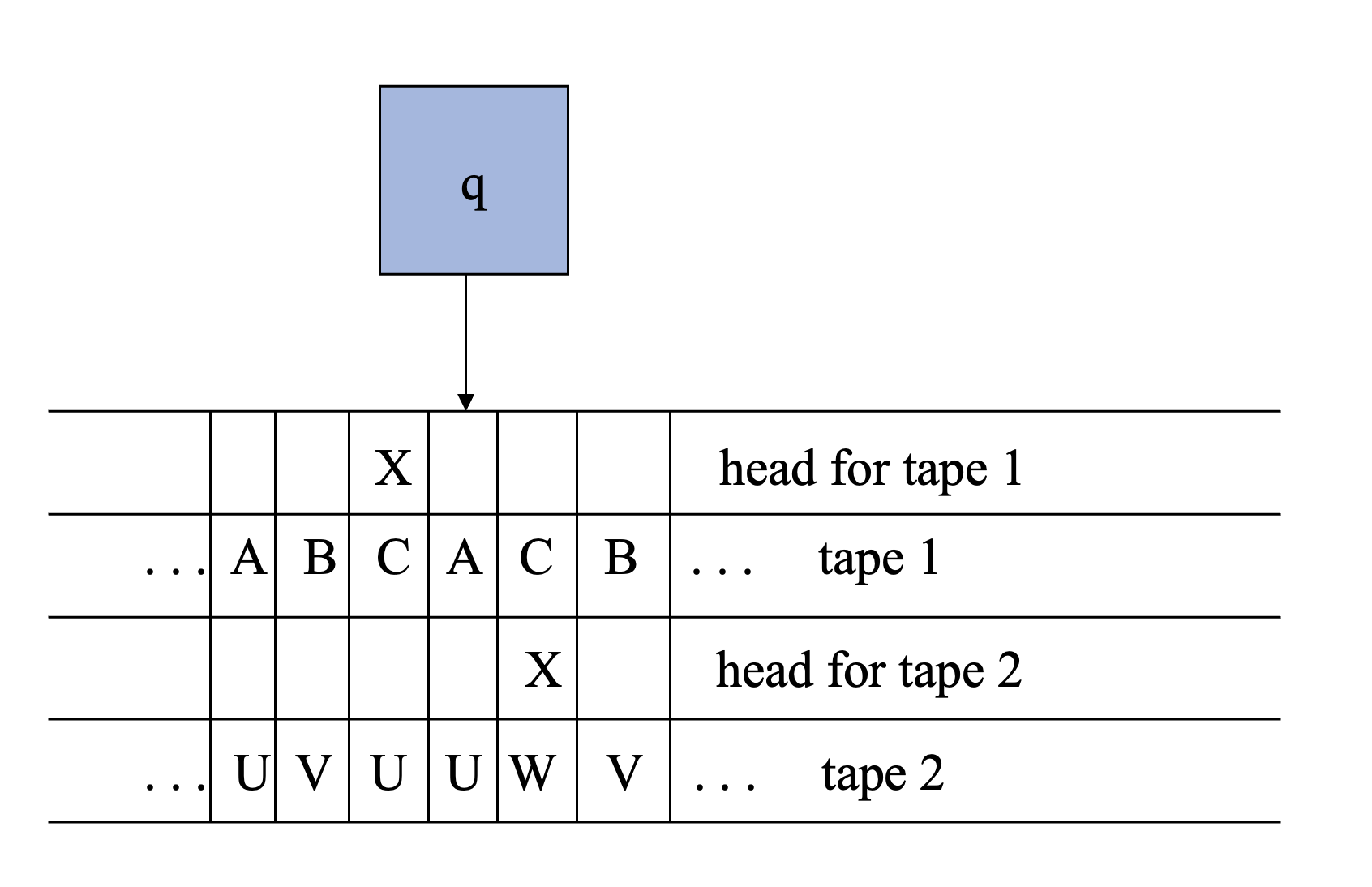
Simulating Multi-Tape TM
Non-deterministic Turing Machine
As NFAs, non-deterministic Turing machines can have multiple transitions for a single state and symbol. The machine accepts the input string if there exists a path that leads to an accepting state.
The non-deterministic Turing machine is equivalent to the deterministic Turing machine. We can convert a non-deterministic Turing machine to a deterministic Turing machine by breadth-first search.
Converting an NTM
We can convert an NTM (non-deterministic Turing machine) to a multi-track DTM by using the idea of BFS. The DTM has two tracks:
- One track records a queue of ID's of the NTM.
- The other track is used to mark certain positions. One mark denotes the current state of the NTM, which is the head of the queue. The other mark help to simulate the transitions of the NTM, adding one-move change to the rear of the queue.
Then, we can use the DTM to simulate the NTM by breadth-first search.
The DTM derived from the NTM does have an upper bound of the size of states and queue. Let the upper bound of numbers of the moving-choices of the NTM be