Finite Automata
Introduction: What is a Finite Automaton?
Finite automaton is a formal system that remembers only a finite amount of information. A finite automaton has the following components:
- Information is stored in the form of states.
- States changes in response to inputs.
- Rules that tell how the states change in response to inputs are called transitions.
Finite automata are widely used for both design and verification of circuits and communication protocols. It is also used in lexical analysis of programming languages, parsing of programming languages, and pattern matching, which makes it a very important component of compilers.
A brief example: Tennis
Basic rules of tennis in a Game:
- One player serves the ball throughout the game.
- To win a game, a player must win at least four points and be ahead by at least two points.
We can model the game of tennis as a finite automaton. The input to the automaton is the sequence of points won by the two players, we name the input alphabet as
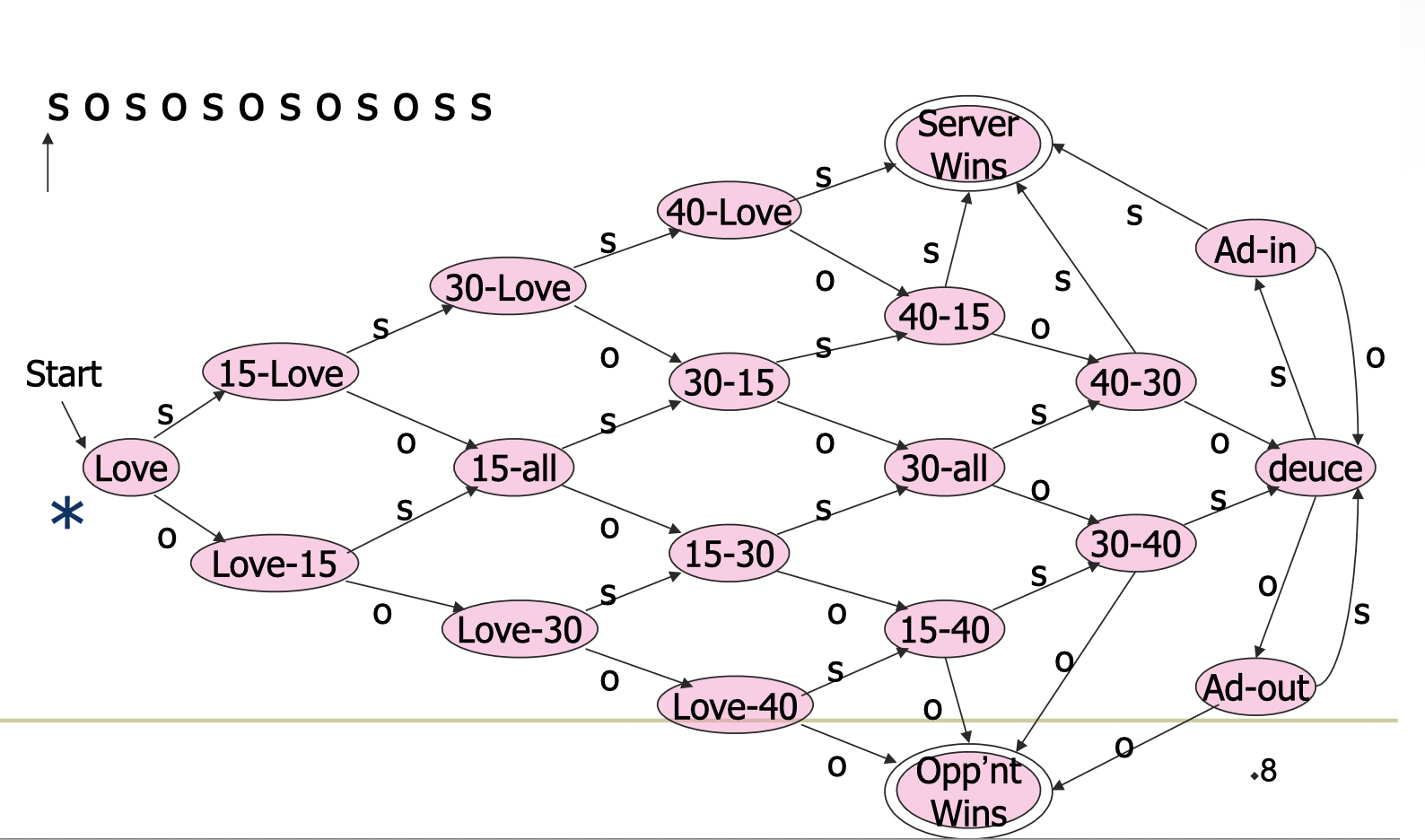
Finite Automaton for Tennis Game
Acceptance of Inputs
Given a sequence of inputs, a finite automaton can either accept or reject the input. The automaton first start in the start state and follows the transitions based on the input sequence. If the automaton reaches one of the accepting states after processing the input, then the input is accepted. Otherwise, the input is rejected.
The set of all inputs that are accepted by the automaton
Deterministic Finite Automaton (DFA)
Preliminary Knowledge: Alphabet, String, Language, Star-Closure, Concatenation, etc.
DFA is a formalism for defining languages, which is consists of the following components:
- A finite set of states
. - A finite set of input alphabet
. - A transition function
. - A start state
. - A set of accepting states (final States)
.
Therefore, a DFA can be defined as a 5-tuple
Transition Function
A total function on
Due to the total function, if there is no transition defined for a state and an input, then the automaton will get stuck and reject the input, also known as dropping into a dead state. Once the automaton reaches a dead state, it will never be able to reach an accepting state.
Graphical and Transition Table Representation
DFA can be represented as a directed graph, where the states are represented as nodes and the transitions are represented as edges. The start state is denoted by an incoming arrow and the accepting states are denoted by double circles.
A... Final state?
Final state can also have transitions to some other states. That is, there can be outgoing edges from the final state. So, how to determine if a string is accepted by the DFA?
The string is accepted by the DFA if and only if the automaton reaches an accepting state and the input is completely processed.
Examples of Graphical Representation of DFA:
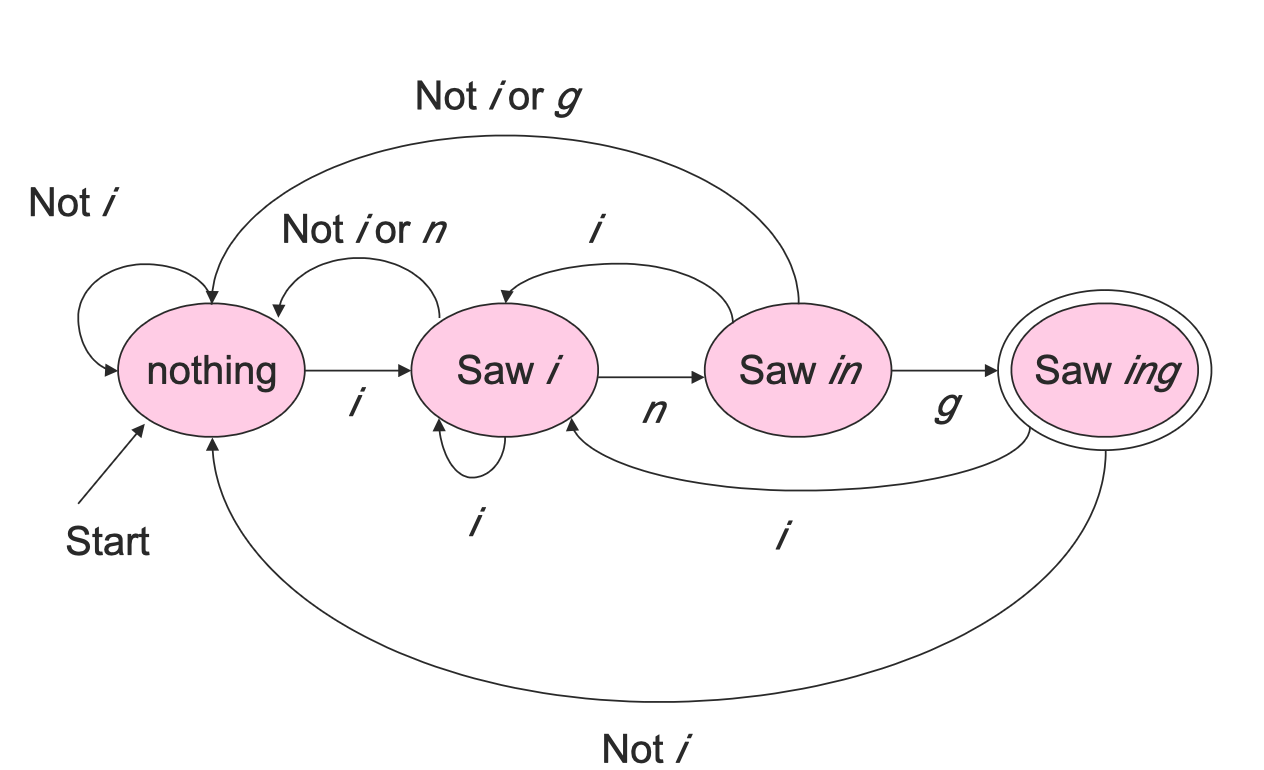
Recognizing String Ending in 'ing'
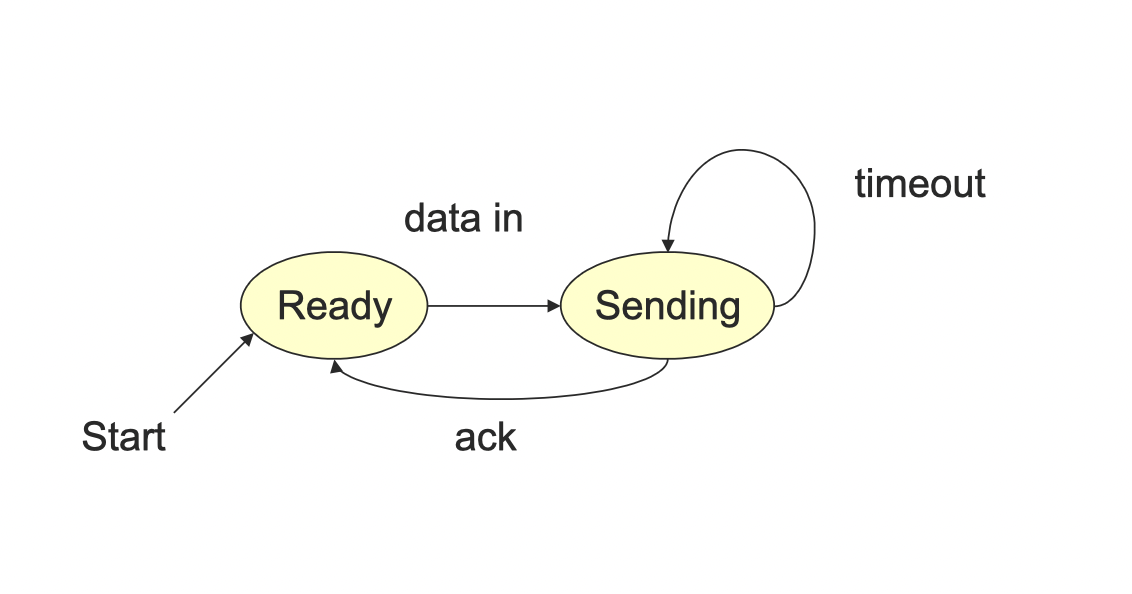
Protocol of Sending Data
DFA can also be represented as a transition table, where the rows represent the states and the columns represent the input alphabet. The transition function is represented as the value in the cell corresponding to the state and input alphabet.
Extended Transition Function
We describe the effect of a string of inputs on a DFA by extending
By using the extended transition function, we can describe the induction on the length of the string:
- Basis:
for all . - Induction:
for all , , and .
Language of a DFA
The language of a DFA
Proof of Language Equivalence
Two DFAs are said to be equivalent if they accept the same language. The usual way to prove the equivalence of two DFAs is to show that their languages are the subset of each other. In order to do that, we often use mathematical induction on the length of the string.
For example, we can prove that the DFA with the language
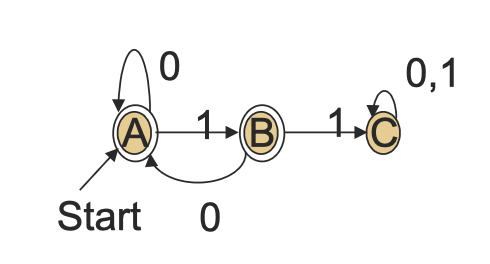
Another DFA's Graphical Representation
Regular Languages
A language
Some languages are not regular. For example, the language
Let us look at some examples of regular languages:
- The language
, which accepts all strings of 0's and 1's. .
Example: A regular Language
Let us consider the language of binary strings that are divisible by
We are going to construct a DFA that recognizes this language. The DFA will have
The start state will be
Since we can construct a DFA that recognizes this language, the language is regular. This example demonstrates that not all regular languages are simple or easy to describe, but they can still be recognized by a DFA.
Nondeterministic Finite Automata (NFA)
A nondeterministic finite automaton (NFA) is a finite automaton that can be in several states at once. The NFA can have multiple transitions from a state on the same input symbol. The NFA accepts a string if there is at least one path that leads to an accepting state.
Just as DFAs, NFAs can be described by a 5-tuple
Apperently, the extended transition function
Extended Transition Function
The extended transition function
- Basis:
for all . - Induction:
for all , , and .
Therefore, we can say the string
NFA with -transitions
An NFA can also have
An example of epsilon-transitions
Next, we will introduce the concept of
If we include
- basis:
for all . - Induction:
for all , , and .
Equivalence of NFAs and DFAs
A DFA can be converted to an NFA that accepts the same language and vice versa.
NFA are easier to design and often have exponentially fewer states than a DFA. Though, only DFA can be implemented!